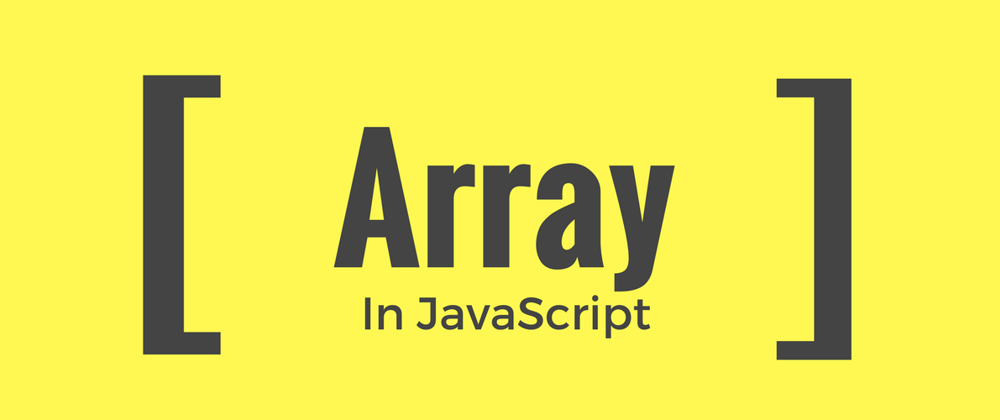
I often ask in interviews: Can you generate a two-dimensional array using JavaScript? This question might seem simple, but it actually reveals the ...
For further actions, you may consider blocking this person and/or reporting abuse
Another way, we can implement
method 3
usingArray.fill()
withoutmap
--UPD:
This version of
create2DArray
usesArray.from
to create a new array for each row, ensuring that each row is independent of the others. Now modifying one row won't affect the others.This is incorrect. You will create an array of multiple copies of the same array:
Indeed, there is this issue. Thumbs up for you!
Thank you for identifying that @jonrandy
This version of
create2DArray
usesArray.from
to create a new array for each row, ensuring that each row is independent of the others. Now modifying one row won't affect the others.This shares a striking resemblance to Method 2 in its ingenuity.
Thank you for your feedback, this is a better approach.
I prefer try it in a way closer to OO paradigme, creating a function that will represent a matrix in general this give to us a more reusefull project feature.
Now we can do:
good job
One more way :)
Nice in depth knowledge of array and js thanks keep it up
Thank you for your support.
Nice article
Thank you for your support.
NB. Did you complete the article word by word or translate it using tools?
Do you have any questions?
Give DaLao a call !!!
You are the best!