{
"name": "merncrud",
"version": "0.1.0",
"private": true,
"dependencies": {
"axios": "^1.3.4",
"bootstrap": "^5.3.3",
"cra-template": "1.2.0",
"formik": "^2.2.9",
"react": "^19.0.0",
"react-bootstrap": "^2.10.6",
"react-dom": "^19.0.0",
"react-scripts": "5.0.1",
"react-toastify": "^10.0.6",
"sweetalert2": "^11.7.0",
"web-vitals": "^4.2.4",
"yup": "^0.32.11"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
},
"eslintConfig": {
"extends": [
"react-app",
"react-app/jest"
]
},
"browserslist": {
"production": [
">0.2%",
"not dead",
"not op_mini all"
],
"development": [
"last 1 chrome version",
"last 1 firefox version",
"last 1 safari version"
]
}
}
`
`import React, {useState, useEffect} from 'react';
import './App.css';
import { Table, Row, Col, Button } from 'react-bootstrap';
import AddModal from './component/AddModal';
import axios from 'axios';
import { ToastContainer, toast } from 'react-toastify';
import Swal from 'sweetalert2';
function App() {
const [show, setShow] = useState(false);
const handleClose = () => setShow(false);
const [rowData, setRowData] = useState([
{firstname: "Punam", lastname: "Khobragade" },
]);
const [rowValues, setRowValues] = useState("")
useEffect(()=>{
fetch('http://localhost:5001/api/users')
.then(response => response.json())
.then(data => setRowData(data));
},[])
const handleShow = () => {
setRowValues("")
setShow(true);
}
const handleDelete = (data) => {
Swal.fire({
title: 'Are you sure?',
text: "You wan't to delete data!",
icon: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6',
cancelButtonColor: '#d33',
confirmButtonText: 'Yes, delete it!',
}).then((result) => {
if (result.isConfirmed) {
fetch(`http://localhost:5001/api/user/${data._id}`, {
method: "DELETE",
body: JSON.stringify({
}),
headers: {
"Content-type": "application/json; charset=UTF-8",
},
})
.then(response => response.json())
.then(data => {
Swal.fire('Deleted!', 'The user has been deleted.', 'success');
fetch('http://localhost:5001/api/users') // api for the get request
.then(response => response.json())
.then(data => setRowData(data));
})
}
});
}
const handleEdit = (data) => {
Swal.fire({
title: 'Are you sure?',
text: "You wan't to Edit data!",
icon: 'warning',
showCancelButton: true,
confirmButtonColor: '#3085d6',
cancelButtonColor: '#d33',
confirmButtonText: 'Yes',
}).then((result) => {
if (result.isConfirmed) {
setRowValues(data);
setShow(true)
}
});
}
const formatDate = (date) => {
if (!date) return '';
const parsedDate = new Date(date);
const year = parsedDate.getFullYear();
const month = (parsedDate.getMonth() + 1).toString().padStart(2, '0');
const day = parsedDate.getDate().toString().padStart(2, '0');
return `${year}-${month}-${day}`;
};
return (
<>
<ToastContainer/>
<Row className="p-5">
<Col md={12} className='d-flex justify-content-between align-items-center'>
<h4>User List</h4>
<span>
<Button variant="info" onClick={(()=>handleShow())}>Add User</Button>
</span>
</Col>
<Col md={12} className='mg-t-15'>
<Table striped bordered hover responsive>
<thead>
<tr>
<th>#</th>
<th>First Name</th>
<th>Last Name</th>
<th>Photo</th>
<th>Gender</th>
<th>Email</th>
<th>Mobile Number</th>
<th>DOB</th>
<th>City</th>
<th>Professional Skills</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
{rowData?.length > 0 && rowData.map((item, index)=>(
<tr key={index}>
<td>{index + 1}</td>
<td>{item?.firstname}</td>
<td>{item?.lastname}</td>
<td><img src={item?.photo} alt="" height={50} width={50}/></td>
<td>{item?.gender}</td>
<td>{item?.email}</td>
<td>{item?.mobile}</td>
<td>{formatDate(item?.dob)}</td>
<td>{item?.city}</td>
<td>{item?.skills}</td>
<td>
<Button variant='info' onClick={(()=>handleEdit(item))}>Edit</Button>
<Button variant = "danger" onClick={(()=>handleDelete(item))}>Delete</Button>
</td>
</tr>
))}
</tbody>
</Table>
</Col>
</Row>
{show &&
<AddModal
show={show}
handleClose={handleClose}
rowValues={rowValues}
setRowData={setRowData}
/>}
</>
);
}
export default App;
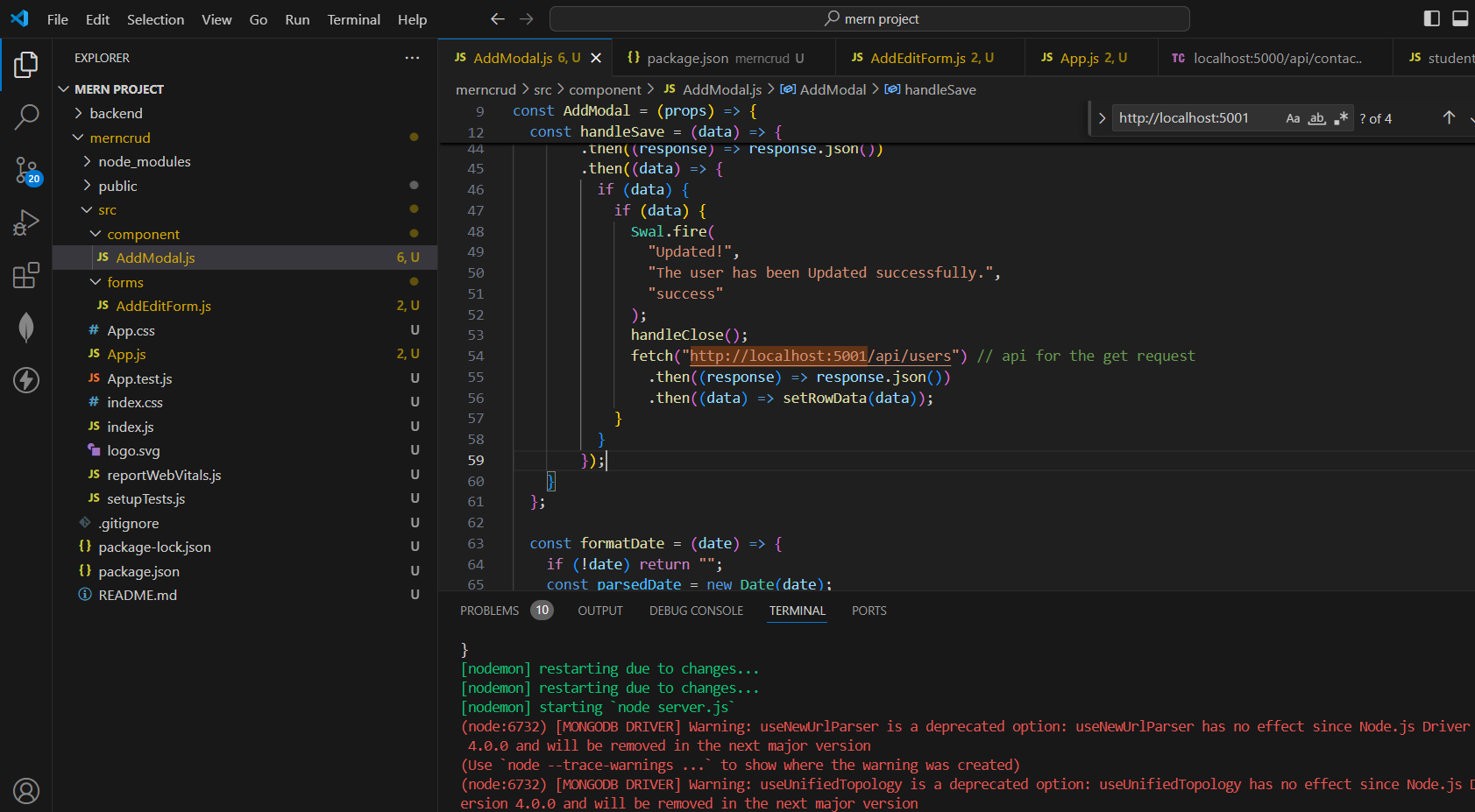`
`import React, { useState } from "react";
import { Form, Button, Modal, Row } from "react-bootstrap";
import { ToastContainer, toast } from "react-toastify";
import { useFormik } from "formik";
import * as Yup from "yup";
import axios from "axios";
import Swal from "sweetalert2";
const AddModal = (props) => {
const { show, handleClose, rowValues, setRowData } = props;
const handleSave = (data) => {
console.log(data, "data1111111111");
if (!rowValues) {
fetch(`http://localhost:5001/api/user/`, {
method: "POST",
body: JSON.stringify(data),
headers: {
"Content-type": "application/json; charset=UTF-8",
},
})
.then((response) => response.json())
.then((data) => {
if (data) {
Swal.fire(
"Created!",
"The user has been Created successfully.",
"success"
);
handleClose();
fetch("http://localhost:5001/api/users")
.then((response) => response.json())
.then((data) => setRowData(data));
}
});
} else {
fetch(`http://localhost:5001/api/user/${rowValues._id}`, {
method: "PUT",
body: JSON.stringify(data),
headers: {
"Content-type": "application/json; charset=UTF-8",
},
})
.then((response) => response.json())
.then((data) => {
if (data) {
if (data) {
Swal.fire(
"Updated!",
"The user has been Updated successfully.",
"success"
);
handleClose();
fetch("http://localhost:5001/api/users") // api for the get request
.then((response) => response.json())
.then((data) => setRowData(data));
}
}
});
}
};
const formatDate = (date) => {
if (!date) return "";
const parsedDate = new Date(date);
const year = parsedDate.getFullYear();
const month = (parsedDate.getMonth() + 1).toString().padStart(2, "0");
const day = parsedDate.getDate().toString().padStart(2, "0");
return `${year}-${month}-${day}`;
};
const addEditUserForm = useFormik({
enableReinitialize: true,
initialValues: {
firstName: rowValues?.firstname || "",
lastName: rowValues?.lastname || "",
email: rowValues?.email || "",
mobile: rowValues?.mobile || "",
photo: rowValues?.photo || "",
gender: rowValues?.gender || "",
city: rowValues?.city || "",
skills: rowValues?.skills ? rowValues.skills.split(",") : [],
dob: rowValues?.dob ? formatDate(rowValues.dob) : "",
},
validationSchema: Yup.object().shape({
firstName: Yup.string().required("Required"),
lastName: Yup.string().required("Required"),
email: Yup.string().required("Required").email("Invalid email address"),
mobile: Yup.string()
.required("Required")
.matches(/^[0-9]{10}$/, "Mobile number must be 10 digits"),
city: Yup.string().required("Required"),
dob: Yup.string().required("Required"),
gender: Yup.string()
.required("Gender is required")
.oneOf(["male", "female"], "Required"),
skills: Yup.array().min(1, "At least one skill is required"),
}),
onSubmit: (values) => {
const skillsData =
values.skills?.length > 0 && values.skills.map(String).join(",");
console.log(values, "dfdfdfdfdf", skillsData);
const payload = {
firstname: values.firstName,
lastname: values.lastName,
email: values.email,
mobile: values.mobile,
photo: values.photo,
gender: values.gender,
city: values.city,
skills: skillsData,
dob: values.dob,
photo: values.photo,
};
handleSave(payload);
},
});
console.log(addEditUserForm, "addEditUserForm");
const handlePhotoChange = (event) => {
const file = event.target.files[0];
if (file) {
const reader = new FileReader();
reader.onloadend = () => {
addEditUserForm.setFieldValue("photo", reader.result);
};
reader.readAsDataURL(file);
}
};
const skillsArray = [
{ label: "Communication", value: "Communication" },
{ label: "Critical Thinking", value: "Critical Thinking" },
{ label: "Problem Solving", value: "Problem Solving" },
];
console.log(addEditUserForm, "addEditUserForm111");
return (
<>
<ToastContainer />
<Modal show={show} onHide={handleClose} size="xl" backdrop="static">
<Form onSubmit={addEditUserForm.handleSubmit}>
<Modal.Header closeButton>
<Modal.Title>{rowValues ? "Update" : "Add"} User</Modal.Title>
</Modal.Header>
<Modal.Body>
<Row>
<Form.Group className="mb-3 col-md-6" controlId="firstName">
<Form.Label>First Name</Form.Label>
<Form.Control
id="firstName"
name="firstName"
type="text"
onChange={addEditUserForm.handleChange}
value={addEditUserForm.values.firstName}
autoComplete="off"
/>
{addEditUserForm.errors.firstName &&
addEditUserForm.touched.firstName ? (
<div className="text-danger">
{addEditUserForm.errors.firstName}
</div>
) : null}
</Form.Group>
<Form.Group className="mb-3 col-md-6" controlId="lastName">
<Form.Label>Last Name</Form.Label>
<Form.Control
id="lastName"
name="lastName"
type="text"
onChange={addEditUserForm.handleChange}
value={addEditUserForm.values.lastName}
autoComplete="off"
/>
{addEditUserForm.errors.lastName &&
addEditUserForm.touched.lastName ? (
<div className="text-danger">
{addEditUserForm.errors.lastName}
</div>
) : null}
</Form.Group>
{console.log(addEditUserForm.values, "fgfgffgf")}
<Form.Group className="mb-3 col-md-6" controlId="dob">
<Form.Label>Date of Birth</Form.Label>
<Form.Control
id="dob"
name="dob"
type="date"
onChange={addEditUserForm.handleChange}
value={addEditUserForm.values.dob || ""}
autoComplete="off"
/>
{addEditUserForm.errors.dob && addEditUserForm.touched.dob ? (
<div className="text-danger">
{addEditUserForm.errors.dob}
</div>
) : null}
</Form.Group>
<Form.Group className="mb-3 col-md-6" controlId="photo">
<Form.Label>Photo</Form.Label>
<Form.Control
id="photo"
name="photo"
type="file"
onChange={handlePhotoChange}
// value={addEditUserForm.values.photo}
/>
{addEditUserForm.errors.photo &&
addEditUserForm.touched.photo ? (
<div className="text-danger">
{addEditUserForm.errors.photo}
</div>
) : null}
</Form.Group>
<Form.Group className="mb-3 col-md-6" controlId="gender">
<Form.Label>Gender</Form.Label>
<div className="d-flex align-items-center">
<Form.Check
type="radio"
id="1"
label="Male"
checked={addEditUserForm.values.gender == "male"}
onClick={() =>
addEditUserForm.setFieldValue("gender", "male")
}
/>{" "}
<Form.Check
type="radio"
id="1"
checked={addEditUserForm.values.gender == "female"}
label="Female"
onClick={() =>
addEditUserForm.setFieldValue("gender", "female")
}
/>
</div>
{addEditUserForm.errors.gender &&
addEditUserForm.touched.gender ? (
<div className="text-danger">
{addEditUserForm.errors.gender}
</div>
) : null}
</Form.Group>
<Form.Group className="mb-3 col-md-6" controlId="formBasicEmail">
<Form.Label>Email Id</Form.Label>
<Form.Control
id="email"
name="email"
type="text"
onChange={addEditUserForm.handleChange}
value={addEditUserForm.values.email}
autoComplete="off"
/>
{addEditUserForm.errors.email &&
addEditUserForm.touched.email ? (
<div className="text-danger">
{addEditUserForm.errors.email}
</div>
) : null}
</Form.Group>
<Form.Group className="mb-3 col-md-6" controlId="formBasicEmail">
<Form.Label>Mobile No.</Form.Label>
<Form.Control
id="mobile"
name="mobile"
type="text"
onChange={addEditUserForm.handleChange}
value={addEditUserForm.values.mobile}
autoComplete="off"
/>
{addEditUserForm.errors.mobile &&
addEditUserForm.touched.mobile ? (
<div className="text-danger">
{addEditUserForm.errors.mobile}
</div>
) : null}
</Form.Group>
<Form.Group className="mb-3 col-md-6" controlId="formBasicEmail">
<Form.Label>City</Form.Label>
<Form.Select
aria-label="Default select example"
onChange={(e) =>
addEditUserForm.setFieldValue("city", e.target.value)
}
value={addEditUserForm.values.city}
>
<option value="*"></option>
<option value="Nagpur">Nagpur</option>
<option value="Chandrapur">Chandrapur</option>
<option value="Mumbai">Mumbai</option>
</Form.Select>
{addEditUserForm.errors.city && addEditUserForm.touched.city ? (
<div className="text-danger">
{addEditUserForm.errors.city}
</div>
) : null}
</Form.Group>
<Form.Group className="mb-3 col-md-12" controlId="formBasicEmail">
<Form.Label>Skills</Form.Label>
<div className="d-flex align-items-center">
{skillsArray?.map((item, index) => (
<span style={{ marginRight: "10px" }} key={index}>
<Form.Check
type="checkbox"
id={index}
label={item?.label}
checked={addEditUserForm.values.skills.includes(
item.label
)}
onChange={() => {
const updatedSkills =
addEditUserForm.values.skills.includes(item.label)
? addEditUserForm.values.skills.filter(
(skill) => skill !== item.label
)
: [...addEditUserForm.values.skills, item.label];
addEditUserForm.setFieldValue(
"skills",
updatedSkills
);
}}
/>
</span>
))}
{addEditUserForm.errors.skills &&
addEditUserForm.touched.skills ? (
<div className="text-danger">
{addEditUserForm.errors.skills}
</div>
) : null}
</div>
</Form.Group>
</Row>
</Modal.Body>
<Modal.Footer>
<Button variant="secondary" onClick={handleClose}>
Close
</Button>
<Button variant="info" type="submit">
Submit
</Button>
</Modal.Footer>
</Form>
</Modal>
</>
);
};
export default AddModal;
Top comments (0)