OTPfy is a brand new product that came out recently. A Software as a Service platform where you will be able to generate One Time Password codes and Magic Links to secure your application flows. Engineering time is valuable, and securing your application flows can be challenging and time consuming. With OTPfy you can secure any part of your application with ease without wasting any more time and resources.
In this post I'll guide you through the steps that will allow you to start securing your application with OTPfy and by the end of the post you'll be familiar with OTP keys, Magic Link keys and the OTPfy SDK which we will make use of via Node.js.
Step 1: Creating a team in OTPfy
Access https://otpfy.com and then click on the sign up
button on the top-right corner. This will take you to the sign up form to create a personal account.
Let's fill the form to create an account with your personal data.
After filling the form, your personal account will be created and we may proceed to creating an OTPfy Team. Click on the Create team
pink button on the top-right corner and it will take you to this form where you will be prompted to fill with your company data. All of this data will be user for billing if you decide to move to a paid license later. All of the teams in OTPfy start with a Free
license which is more than enough to start working on your application and securing your flows while developing. Later on, you can move to a paid license to increase the limits for team as needed.
After filling the form, your team will be ready and will redirect you to your Dashboard and you can start working with OTPfy. Let's take a look at your team's Dashboard on the step 2.
Step 2: Dashboard overview
This is your team's Dashboard. Here you can get an overview on how your OTPs and Magic Links are behaving and a quick overview on the team's license usage.
Let's describe the navigation menu from left to right:
- Dashboard: get your team's overview & insights.
- Team: in this page you will be able to update your team details, add and remove members from your team and your personal notifications. In OTPfy, notifications are handled on a per team basis, meaning that you can customize the notifications you'd like on each team independently.
- Keys: this is the page where you will generate OTP keys and Magic Link keys. This keys are the angular stone of OTPfy, from this keys you will generate OTP codes and Magic Links for your users, applications and flows.
- Templates: here you will be able to generate and configure the templates that will be sent to the user that request an OTP or a Magic Link. Templates are fully customizable with HTML, you can use the default one or get creative.
-
License: this page will display you current team's license (Free & unlimited) and you will be able to upgrade to any of the paid licenses:
PRO
,STARTUP
orBUSINESS
. After subscribing you'll be able to cancel your license at period end or if you have cancelled it, enable it back. Remember that all of the annual licenses comes with a 20% OFF! Also on this page you will be able to view your generated invoices or pay due invoices. - Payment Details: here you will be able to update your payment details in case you need it due to credit card expiration or something related.
Profile dropdown navigation
- Account Settings: update your personal information like picture, first name, last name and phone.
- Change team: select a different team you're in to operate with.
- Support: access the support system to report any issue you might encounter (hoping you don't have to use this much!)
- Sign out: close your session at OTPfy.
Step 3: Creating a template for an OTP key.
The first thing you'd need to do is to create a template. Let's get started with it! Go to the Templates
page and click on the Create template
pink button that appears on the right side of the page, this will display a modal window with the following fields to fill:
After the creation process finished, you'll be taken to the Template Edition
page where you'll be able to update the previous details and get access to the HTML template editor. You an also have a preview of what your HTML template will look like!
Step 4: Creating an OTP key
Next thing to do is to create an OTP key, this key will allow you to generate an OTP for securing a flow with the configuration that you set on this key. Click on the Keys
navigation menu to get to the Keys
page where you can have a general overview on how your keys for OTPs
and Magic Links
are behaving. Click on the OTP
secondary navigation element. You'll se an empty page with a nice illustration telling you that you don't have any OTP key created yet. Let's click on the Add OTP key
pink button on the right side of this page. This action will display a modal window with a form where you can configure how this OTP key will behave:
Let's get into details about the configurations:
- Key identifier: this key identifier is what you'll use on the OTPfy SDK to tell which OTP key is going to be used and it will take all of the configuration from.
- Name: the key name for identification purposes on the Dashboard.
- Description: the key description for identification purposes on the Dashboard.
- Template: here you will select the template that we have previously created on the Step 3.
- Code length: the amount of characters long this code will be, you can input any number between 6 and 24.
- Expiry time (in seconds): the amount of seconds that the OTP will be valid after its generation, you can input any number of seconds between 60 and 300.
- Color: the key color for identification purposes on the Dashboard.
-
Additional configurations:
- Use numbers: this setting will tell OTPfy to include numbers on the OTP generated.
- Use uppercase characters: this setting will tell OTPfy to include uppercased characters on the OTP generated.
- Use special characters: this setting will tell OTPfy to include special characters on the OTP generated.
After you've finished setting up the data, click on the Add OTP key
button to generate this key and be able to use it via OTPfy SDK.
If you're curious, you can click on the More details
button of your brand new OTP key
to get specific details on how this OTP key
is behaving.
Step 5: Obtaining your team's private key
If you navigate to your Team
page and access the Details
tab, you'll see at the bottom of the details a section that reads Private key
. To reveal your private key click on the Reveal private key
white button at the right side of this row, this will show your unique and exclusive team's private key, if you click on the button again, it will be automatically copied to your clipboard.
Step 6: Installing the OTPfy SDK
The next step is installing the OTPfy SDK in your Node.js application (currently we only have published the Node SDK but we're working hard on getting more SDKs out for any of your backend languages!).
Access you project via console and type:
yarn add @otpfy/sdk
This will install the OTPfy SDK in your backend application. This module requires the use of node-fetch
as a dependency but it's not bundled with the SDK to avoid increasing the bundle size. This means that you will have to separately install node-fetch
as a dependency of your backend project.
Step 7: SDK initialization
In order for a successful initialization your team private key needs to be passed as the only argument to the initialization function. Make sure that this private key is always safe and secure so nobody can make requests on your team's behalf.
import sdk from '@otpfy/sdk';
const otpfy = sdk('TEAM_PRIVATE_KEY');
Step 8: OTP generation
Access to any flow of your application where you'd like to generate the OTP and paste the following code that you will modify a little.
const generateResponse = await otpfy.generateOTP({
email: 'me@gmail.com',
external_identifier: '978c324ab78dd92',
key: 'AUTH'
});
This code will tell OTPfy to generate an OTP with the following configuration:
- email: recipient email of the OTP that will be generated.
- external_identifier: unique ID that you must provide to uniquely identify this OTP for a particular user or flow. Commonly, this will be, as per this example, the user identifier that's generated on your database.
- key: the key identifier that you have previously used on the OTP key generation. This will take all of the configurations that you've previously setup.
The generateResponse
variable will be an object with the following shape:
type GenerateResponse = {
status: number;
code: string;
identifier: string;
message?: string;
};
```
The `status` property will contain the status code of the operation which might be 200 if the success was `OK` or `400` to `500` depending on the error that occurred.
The `code` property will contain a message form OTPfy telling what happened, might contain a message like `SUCCESS` if everything went right or `BAD_REQUEST` or `UNEXPECTED_ERROR` depending on what caused it.
The `identifier` property is what you must store to later on verify this OTP that you have just generated.
The `message` property will be present if the status is different from 200 and will tell you a little more information about what have caused the error.
# Step 9: OTP verification
Access to any flow of your application where you'd like to check for the OTP was received from your user and paste the following code that you will modify a little.
```typescript
const verifyResponse = await otpfy.verifyOTP({
code: '45$6AFd65',
external_identifier: '978c324ab78dd92',
key: 'AUTH',
identifier: '123cb8945bd0943'
});
```
This code will tell [OTPfy](https://otpfy.com) to verify an OTP with the following configuration:
* **code**: the code that the user have received via email.
* **external_identifier**: Unique identifier provided on your side to identify this Magic Link. Commonly this could be your user's ID or some unique identifier.
* **key**: the _key identifier_ that you have previously used on the OTP key generation. This will take all of the configurations that you've previously setup.
* **identifier**: the identifier that was returned from the `otpfy.generateOTP` function.
The `verifyResponse` variable will be an object with the following shape:
````typescript
type VerifyResponse = {
status: number;
code: string;
message?: string;
};
```
{% endraw %}
The {% raw %}`status`{% endraw %} property will contain the status code of the operation which might be 200 if the success was {% raw %}`OK`{% endraw %} or {% raw %}`400`{% endraw %} to {% raw %}`500`{% endraw %} depending on the error that occurred.
The {% raw %}`code`{% endraw %} property will contain a message form OTPfy telling what happened, might contain a message like {% raw %}`SUCCESS`{% endraw %} if everything went right or {% raw %}`BAD_REQUEST`{% endraw %} or {% raw %}`UNEXPECTED_ERROR`{% endraw %} depending on what caused it.
The {% raw %}`message`{% endraw %} property will be present if the status is different from 200 and will tell you a little more information about what have caused the error.
If the {% raw %}`verifyResponse.status`{% endraw %} is equal to {% raw %}`200`{% endraw %} will mean that the authenticity of the user and the code and the process was a success and you can grant access that person to whatever they were trying access safely.
# Step 10.1: Creating a Magic Link key (optional)
A Magic Link key key will allow you to generate a Magic Link for securing a flow with the configuration that you set on this key. Click on the {% raw %}`Keys`{% endraw %} navigation menu to get to the {% raw %}`Keys`{% endraw %} page where you can have a general overview on how your keys for {% raw %}`OTPs`{% endraw %} and {% raw %}`Magic Links`{% endraw %} are behaving. Click on the {% raw %}`MAgic Link`{% endraw %} secondary navigation element. You'll se an empty page with a nice illustration telling you that you don't have any Magic Link key created yet. Let's click on the {% raw %}`Add Magic Link` pink button on the right side of this page. This action will display a modal window with a form where you can configure how this Magic Link key will behave:
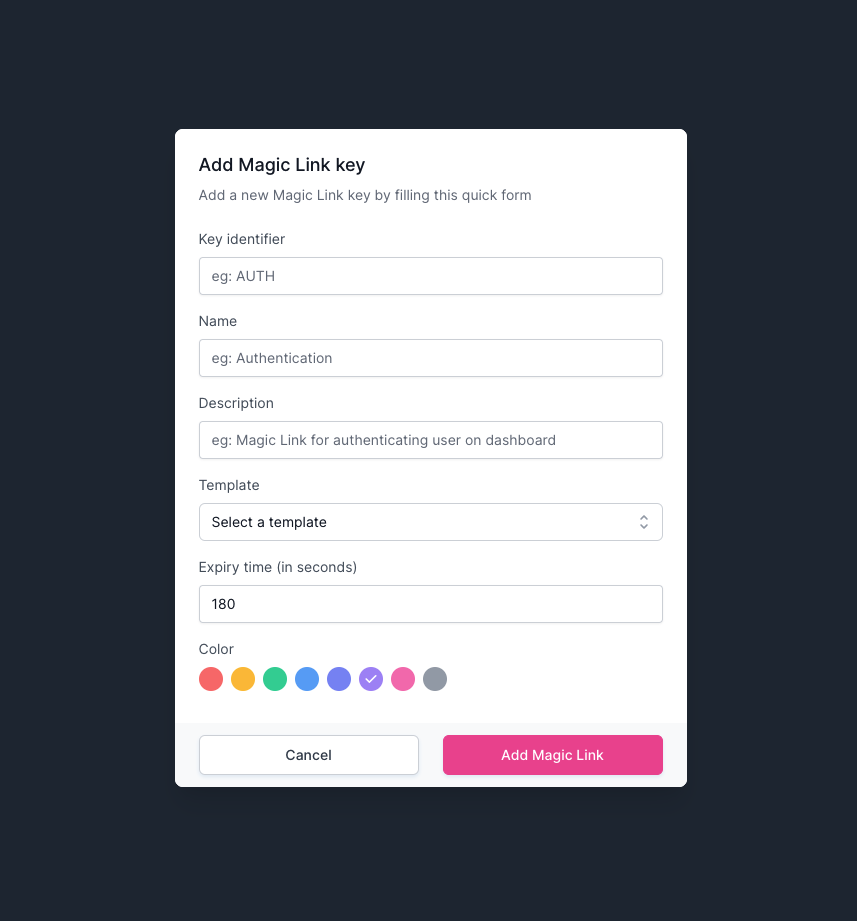
Let's get into details about the configurations:
* **Key identifier**: this key identifier is what you'll use on the [OTPfy SDK](https://otpfy.com/docs/node) to tell which Magic Link key is going to be used and it will take all of the configuration from.
* **Name**: the key name for identification purposes on the Dashboard.
* **Description**: the key description for identification purposes on the Dashboard.
* **Template**: here you will select the template that we have previously created on the Step 3.
* **Expiry time (in seconds)**: the amount of seconds that the Magic Link will be valid after its generation, you can input any number of seconds between 60 and 300.
* **Color**: the key color for identification purposes on the Dashboard.
After you've finished setting up the data, click on the {% raw %}`Add Magic Link`{% endraw %} button to generate this key and be able to use it via [OTPfy SDK](https://otpfy.com/docs/node).
If you're curious, you can click on the `More details` button of your brand new `Magic Link key` to get specific details on how this `Magic Link key` is behaving.
# Step 10.2: Magic Link generation (optional)
In order to generate a new Magic Link for securing a particular flow, you will need to import the [OTPfy SDK](https://otpfy.com/docs/node), initialize it and then call the `optfy.generateMagicLink` method.
```typescript
import sdk from '@otpfy/sdk';
const otpfy = sdk('TEAM_PRIVATE_KEY');
const generateResponse = await otpfy.generateMagicLink({
email: 'me@gmail.com',
external_identifier: '978c324ab78dd92',
key: 'AUTH',
callbacks: {
success: 'https://myapp.com/authentication/success?id=123123123'
error: 'https://myapp.com/authentication/error?id=123123123'
}
});
```
This code will tell [OTPfy](https://otpfy.com) to generate a Magic Link with the following configuration:
* **email**: An email will be sent to this target email with the generated Magic Link based on the template created and chosen on your OTPfy dashboard.
* **external_identifier**: Unique identifier provided on your side to identify this Magic Link. Commonly this could be your user's ID or some unique identifier.
* **key**: the _key identifier_ that you have previously used on the Magic Link key generation. This will take all of the configurations that you've previously setup.
* **callbacks**: this 2 properties will be used to redirect the user to specific parts of your application if the verification has succeeded or failed.
* **success**: URL where the user will be redirected if the Magic Link verification was successful.
* **error**: URL where the user will be redirected if the Magic Link verification was wrong.
Generating Magic Links with [OTPfy](https://otpfy.com) is very straightforwards, and you can even customize the callbacks to pass any data that you'd require on your end when redirecting the user (like the user id that tried to verify or anything else).
# Step 11: Checking your team's license with the SDK (optional)
You can use this handy method from the OTPfy SDK in order to check your team's current license status.
```typescript
const licenseStatus = await otpfy.checkLicense();
```
`licenseStatus` will hold a string literal with any of the following values and meanings:
* `VALID_LICENSE`: Your current license is valid and you can make use and have access to any of the [OTPfy](https://otpfy.com) services.
* `UNAUTHORIZED` The provided team private key at the initialization of the SDK was not valid and therefore, you're not authorized to check for license statuses.
* `TEAM_BLOCKED`: Your team is currently blocked from using the [OTPfy](https://otpfy.com) services due to an unpaid overdue invoice. Please, access your dashboard at https://otpfy.com/license and proceed with the payment of the invoice in order to unblock the license and continue using the [OTPfy](https://otpfy.com) services.
I hope that you all enjoyed the read and start securing your application flows with [OTPfy](https://otpfy.com)!
Top comments (0)