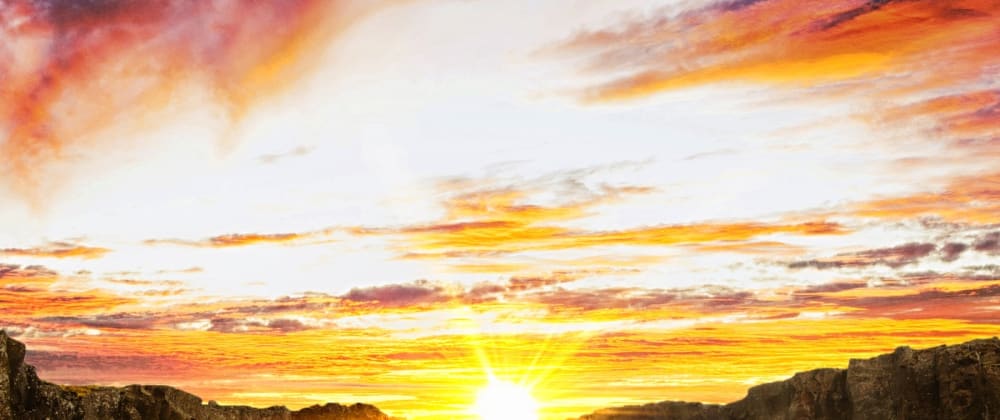
Follow me on Twitter, happy to take your suggestions on topics or improvements /Chris
You may be completely new to JavaScript or you may have onl...
For further actions, you may consider blocking this person and/or reporting abuse
Without the spread operator, you can do:
I kind of prefer that way, since it doesn't involve extra syntax. But I'm used to using the spread operator now.
The fact that this results in an out-of-order array called 'secondHalf' that has ALL the values on it is still annoying...but that's OPs fault not yours @randall
const fullArray = [].concat(firstHalf, secondHalf);
Thanks for the article!
One correction:
find() actually returns
undefined
if no value is matched in the array.good items here
Great post with short and concise examples!
I'd like to add one more example for array destructuring. You can use the fact that arrays are objects and the array indices are object keys. That means with key 0 you get the fist element, but more importantly with a computed property key like [length-2] you can get an element in the middle of the array which is not possible with array destructuring and rest parameters:
codesandbox.io/s/fancy-dust-24cwhr...
This seems like one of those things thats neat, but actually using it in practice will just result in some weird behavior thats difficult to track down...
Or that could just be my inner-old-man
Extracting elements at the beginning of the array should be should be equivalent using array or object destructuring, because if the index does not exist the element is a going to be undefined in both cases: codesandbox.io/s/unruffled-babbage...
And if you want to extract only the last element (not possible using array destructuring), you have to use the length to calc the last index in the way as a direct array index access via brackets: codesandbox.io/s/elated-tdd-6nte4r...
Nevertheless, I wouldn't recommend object destructuring over array destructuring in all cases, but knowing about it shouldn't hurt either.
Great post! 5th & 10th points were my major take away! Thanks!
I don't know if this would be helpful.
For defining constants, instead of regular object,
define constants with
Object.freeze()
.This way, you won't be able to change the entries accidentally.
Happy Coding π§‘
The good news for me at least, is that I use them all!
. + const and let.
nice post!
Good one
Some good tips in here πβ¨π―
Nice job! The only thing that you might reconsider to change if you do is the async/await example. When fetching multiple data from different api's, you should use promise.all instead of async so you can fetch all the data at once instead of waiting for the first one to be finished and to start the second fetch and so on.
Not really. If you look at the example he uses the return value of the first await for the second one, so he can't send them in parallel. It's exactly the use case for async/await :)
If there are no dependencies, I'd agree to use promises and a promise.all()
~Cheers
New things to learn thankyou for sharing
Good post full of practical examples thanks for sharing it with the community.
Thank you for the article, straight to the point with really good examples. Loads of stuff to learn and implement.
Thank you for sharing, I've learned a lot.
I don't think your NO spread for objects is right, I would likely do it like
hi Mike, are you saying this code should use Object.assign()?
Because my point was to showcase how painful it can be without spread? Help me understand, thanks :)
I get that, but just because you can do something more painfully doesn't mean that it can't be done simpler. On top of that, there is no assignment of the weapon key.
I think it can be argued that
{ ...hero, weapon: "sword"}
is easier to read thanObject.assign({}, hero, {weapon:"sword")
yes I agree that spread is easier to read than Object.assign().. so are you saying that it doesn't have to be as painful as looping through the keys but rather Object.assign is there and if you want to improve on that use spread syntax?
I think that is fair to say. Since prior to browser support that was what the transpilers often did for you.
I was thinking exactly this.
Even better: with this approach, you can choose to merge elements into an pre-existing object which can help with keeping object references and reduces the need for the engine to garbage collect short-lived objects.
Feels like a better approach!
looks like a great article for 2015)
you don't agree with the advice?
I can really believe that there are times when, due to an outdated environment, developers could not install babel for themselves all these 7 years. But I have not seen developers who have not changed jobs for 7 years. Usually it is 1-4 years, and then interesting offers on the labor market tell you to change jobs
Hehe totally my thoughts. ( I know, bad me bad me)
Now that IE is retired, it looks like the IE team started writing JS articles
This is a good article in the sense that nothing totally new or ground breaking but some excellent explanations and examples. Thank for sharing.
It's very useful!
The destructuring argument is unconvincing: the "old fashioned" code is extremely clear, easier to update if the structure of the object changes, and allows one to change the variable name, if needed, for even more clarity, e.g.
const nameNeedsValidation = req.param.name
.As others have noted, the Object Spread comment about needing looping is wrong:
Object.assign()
does the job nicely. IMO, it is also clearer than using spread, but I admit that's just my opinion.Destructuring like so:
const { name: nameNeedsValidation } = req.param
Creates a variable called
nameNeedsValidation
from the propertyreq.param.name
.I find it preferable to destructure in the case of when I'm working on a React class component and the state object is complex (10+ properties).
In the template, instead of having to write:
I can just write:
Note that this would be unconventional:
React class components are "old school" now I think, but destructuring is convenient any time you have to work with a complex object in which the properties have to referenced multiple times. That's the use case I'm most familiar with.
Merging objects using spread operator does not make a deep copy. Be very careful with this or you'll really mess up
Quite a helpful post and helps in doing stuff in a less painful way
Last one you could use just .reduce and something like: return [1,2,3].reduce((acc,e)=>acc+e,0)
Going to be one of my favourites. Nice !
Thanks π
I believe the spread object and rest parameter shouldn't be used. source code will be hard to maintain and become out of control when used so much
Very clear explanation thanks!