1. Shorthand for if with multiple OR(||) conditions
if (car === 'audi' || car === 'BMW' || car === 'Tesla') {
//code
}
In a traditional way, we used to write code in the above pattern. but instead of using multiple OR conditions we can simply use an array and includes. Check out the below example.
if (['audi', 'BMW', 'Tesla', 'grapes'].includes(car)) {
//code
}
2. Shorthand for if with multiple And(&&) conditions
if(obj && obj.tele && obj.tele.stdcode) {
console.log(obj.tele .stdcode)
}
Use optional chaining (?.) to replace this snippet.
console.log(obj?.tele?.stdcode);
3. Shorthand for checking null, undefined, and empty values of variable
if (name !== null || name !== undefined || name !== '') {
let second = name;
}
The simple way to do it is...
const second = name || '';
4. Shorthand for switch case to select from multiple options
switch (number) {
case 1:
return 'Case one';
case 2:
return 'Case two';
default:
return;
}
Use a map/ object
const data = {
1: 'Case one',
2: 'Case two'
};
//Access it using
data[num]
5. Shorthand for functions for single line function
function example(value) {
return 2 * value;
}
Use the arrow function
const example = (value) => 2 * value
6. Shorthand for conditionally calling functions
function height() {
console.log('height');
}
function width() {
console.log('width');
}
if(type === 'heigth') {
height();
} else {
width();
}
Simple way
(type === 'heigth' ? height : width)()
7. Shorthand for To set the default to a variable using if
if(amount === null) {
amount = 0;
}
if(value === undefined) {
value = 0;
}
console.log(amount); //0
console.log(value); //0
Just Write
console.log(amount || 0); //0
console.log(value || 0); //0
Top comments (15)
On 6th example i would rather use it in that way
type === 'heigth' ? height() : width()
it is more clear for me and for other i guess, and can be passed different arguments to functions
I agree. It also makes it easier to do a global search for all places where those functions are invoked.
7th example can be written with ??. The difference is that values that evaluate to
false
would pass but if you use||
they don'tExample:
Be careful if you refactor switch by object, because switch uses strict uses strict comparison
===
.console.log(data['1']);
will return 'Case one' butswitch('1')
will jump to the default branch.The 7th example is not entirely correct though.
You replace
with
But if amount is
undefined
, it will not enter your if, but stillundefined || 0
returns0
.In short, you can't replace
=== null
with the||
operator as the behaviour is slightly different.thank for share with us :)
Cheers
'Audi,BMW,Tesla,Daf,Volkswagen'.includes(car);
or even without ,
this would be a good trick if used just on typescript where car has to have enum type that allows only mentioned car brands
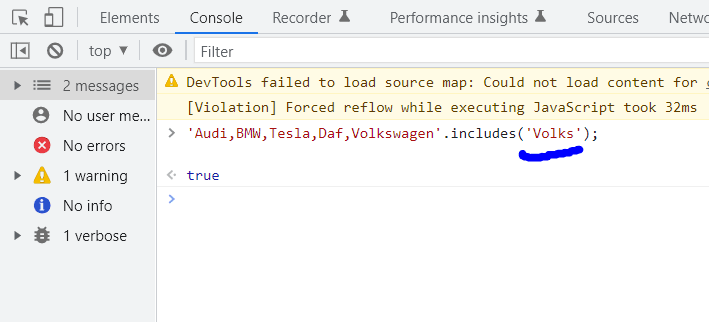
and here is why ( counter example on js )
undefined
but withif
case it won't fire;good reminders always easy to forget optional chaining!
2nd Example optional chaining will return undefined if console.log like that, you would probably need to do use a ternary instead if you didn't want to print undefined.
but thanks for this.
Good examples - thank you. 🙌