In the fast-paced world of financial technology (fintech), the need for reliable, scalable, and real-time communication between services is critical. RabbitMQ, Apache Kafka, and ActiveMQ are three of the most widely used messaging systems, each offering unique advantages suited for different fintech use cases. In this article, we will explore these systems with practical, fintech-related examples, discuss common challenges, and provide actionable solutions to overcome them.
What Are Messaging Systems in Fintech?
Messaging systems allow different services and components in a fintech application to communicate asynchronously. This is essential when dealing with transactions, payments, real-time data streaming, and account updates across multiple systems. Messaging systems ensure reliable, scalable, and decoupled communication, much like how banks and payment gateways exchange data securely without direct real-time connections.
1. RabbitMQ: Use Case – Real-Time Payment Processing
Use Case:
In fintech applications, payment gateways and transaction systems need to process payments in real-time. When a customer initiates a payment, the system must verify the payment method, debit the account, and notify various services (e.g., fraud detection, transaction logging, notification services) of the payment’s status.
RabbitMQ is ideal for handling this type of real-time payment processing where messages need to be delivered reliably and immediately to multiple services.
Common Challenges with RabbitMQ in Fintech
Message Acknowledgment Delays: If consumers take too long to acknowledge payment processing messages, it can cause bottlenecks in the system.
Solution: Use manual acknowledgments combined with prefetch limits to control the number of unacknowledged messages, ensuring that consumers process payments efficiently.
channel.BasicQos(0, 10, false); // Limits the number of unacknowledged messages
Scaling Consumers: As the number of transactions increases, RabbitMQ can experience difficulties in scaling the number of consumers handling payments.
Solution: Implement a RabbitMQ cluster and use multiple consumer instances to horizontally scale payment processing services.
Practical Code Example: RabbitMQ in Payment Processing
Producer code (initiating a payment):
var factory = new ConnectionFactory() { HostName = "localhost" };
using var connection = factory.CreateConnection();
using var channel = connection.CreateModel();
channel.QueueDeclare(queue: "payment_queue", durable: true, exclusive: false, autoDelete: false, arguments: null);
string paymentMessage = "Payment initiated for $100";
var body = Encoding.UTF8.GetBytes(paymentMessage);
channel.BasicPublish(exchange: "", routingKey: "payment_queue", basicProperties: null, body: body);
Console.WriteLine("Payment sent: {0}", paymentMessage);
Consumer code (processing the payment):
using RabbitMQ.Client.Events;
channel.QueueDeclare(queue: "payment_queue", durable: true, exclusive: false, autoDelete: false, arguments: null);
var consumer = new EventingBasicConsumer(channel);
consumer.Received += (model, ea) =>
{
var body = ea.Body.ToArray();
var message = Encoding.UTF8.GetString(body);
Console.WriteLine("Processing payment: {0}", message);
channel.BasicAck(ea.DeliveryTag, false); // Acknowledge the message
};
channel.BasicConsume(queue: "payment_queue", autoAck: false, consumer: consumer);
2. Kafka: Use Case – Transaction Stream Processing for Fraud Detection
Use Case:
In fintech, fraud detection systems rely on analyzing vast amounts of transaction data in real-time. Kafka is well-suited for stream processing due to its high throughput and ability to handle real-time data streams. Transactions from different services are published to Kafka topics and processed in real-time to detect anomalies, suspicious behavior, or fraud patterns.
Kafka is perfect for large-scale transaction monitoring where every transaction must be logged, processed, and analyzed to flag potential fraud.
Common Challenges with Kafka in Fintech
Message Loss: In high-volume transaction systems, improper configuration can result in lost transaction messages.
Solution: Use acks to ensure messages are acknowledged by brokers before being considered successfully produced. Set acks=all for guaranteed delivery.
var config = new ProducerConfig { BootstrapServers = "localhost:9092", Acks = "all" };
Consumer Lag: In high-throughput environments, consumers might not keep up with the stream of transaction data, leading to delays in fraud detection.
Solution: Use consumer groups to distribute the load across multiple consumers and partition transactions by account or region to parallelize processing.
Practical Code Example: Kafka for Fraud Detection
Producer code (publishing transactions for fraud detection):
var config = new ProducerConfig { BootstrapServers = "localhost:9092" };
using var producer = new ProducerBuilder<Null, string>(config).Build();
await producer.ProduceAsync("transaction_stream", new Message<Null, string> { Value = "Transaction of $500 from account 12345" });
Consumer code (processing transactions for fraud analysis):
var config = new ConsumerConfig { GroupId = "fraud_detection_group", BootstrapServers = "localhost:9092", AutoOffsetReset = AutoOffsetReset.Earliest };
using var consumer = new ConsumerBuilder<Null, string>(config).Build();
consumer.Subscribe("transaction_stream");
while (true)
{
var consumeResult = consumer.Consume();
Console.WriteLine("Analyzing transaction: " + consumeResult.Message.Value);
}
3. ActiveMQ: Use Case – Loan Application Processing
Use Case:
In a fintech platform, loan applications are processed by multiple teams: verification, risk assessment, and approval. Each stage of the loan approval process might take time, and the system needs to ensure reliable delivery of loan application data between each department. ActiveMQ excels in such scenarios by ensuring reliable message delivery with support for multiple messaging protocols.
**ActiveMQ **works well for handling reliable, asynchronous communication in workflows that have multiple stages, such as loan processing systems.
Common Challenges with ActiveMQ in Fintech
Slow Message Processing: In a high-volume environment, consumers might process loan applications too slowly, leading to delays.
Solution: Use concurrent consumers and optimize thread pools to process multiple loan applications in parallel.Broker Failures: ActiveMQ brokers may fail under heavy loads, leading to system downtime.
Solution: Implement ActiveMQ clustering or a failover strategy to ensure that messages are always processed, even in the event of a broker failure.
Practical Code Example: ActiveMQ in Loan Application Processing
Producer code (sending loan applications):
using Apache.NMS;
using Apache.NMS.ActiveMQ;
var factory = new ConnectionFactory("tcp://localhost:61616");
using var connection = factory.CreateConnection();
using var session = connection.CreateSession();
var destination = session.GetQueue("loan_queue");
using var producer = session.CreateProducer(destination);
var message = session.CreateTextMessage("Loan Application: John Doe, $10,000");
producer.Send(message);
Console.WriteLine("Sent loan application: {0}", message.Text);
Consumer code (processing loan applications):
using Apache.NMS;
using Apache.NMS.ActiveMQ;
var factory = new ConnectionFactory("tcp://localhost:61616");
using var connection = factory.CreateConnection();
using var session = connection.CreateSession();
var destination = session.GetQueue("loan_queue");
using var consumer = session.CreateConsumer(destination);
var message = consumer.Receive() as ITextMessage;
Console.WriteLine("Processing loan application: {0}", message.Text);
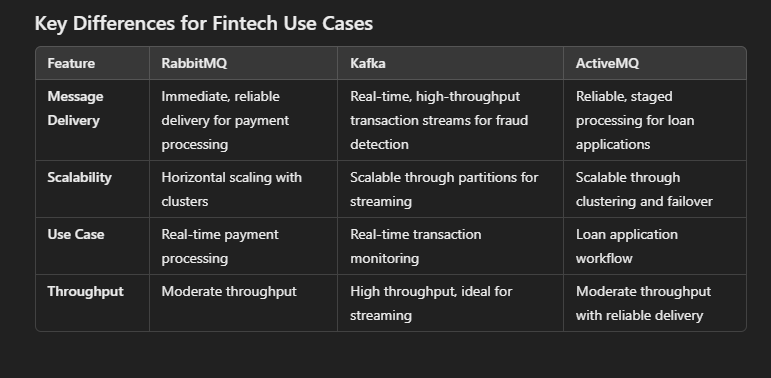
Common Challenges and Solutions in Fintech Messaging Systems
Scalability: In high-volume fintech applications, scalability is crucial to handle millions of transactions or payment events.
Solution: For RabbitMQ, use clustering and increase the number of consumer instances. In Kafka, scale by partitioning topics. For ActiveMQ, ensure clustering and failover configurations are in place.Message Loss: Losing a message in fintech is critical, especially if it’s a payment or transaction detail.
Solution: Configure proper acknowledgment and durability settings. For RabbitMQ and ActiveMQ, ensure message persistence. In Kafka, use acks=all to guarantee delivery.Consumer Performance: Slow consumer processing can delay the handling of transactions or loan approvals.
- Solution: Use concurrent consumers, fine-tune prefetch settings in RabbitMQ, use consumer groups in Kafka, and configure thread pools in ActiveMQ for faster processing.
Monitoring and Alerts: Issues such as consumer lag, message loss, or slow performance need to be detected early.
- Solution: Implement monitoring tools like Prometheus, Grafana, and Datadog to track system health and receive alerts when issues arise.
Conclusion
In fintech, choosing the right messaging system—RabbitMQ, Kafka, or ActiveMQ—depends on your specific use case:
- RabbitMQ is ideal for real-time payment processing, where message reliability and immediate delivery are critical.
- Kafka is perfect for fraud detection and transaction monitoring through high-throughput, real-time streaming.
- ActiveMQ works well in workflows like loan processing, where reliable delivery is essential across multiple stages.
Understanding the challenges and knowing how to configure these systems ensures a smooth, reliable messaging experience in fintech applications.
LinkedIn Account
: LinkedIn
Twitter Account
: Twitter
Credit: Graphics sourced from RST Software
Top comments (0)