iwant-style library imports ready styles using CSS in JS to be used in conjunction with styled-components.
Screenshot

Contents
Getting Start
npm install styled-components
Usage
import styled from 'styled-components';
import { inputDark } from 'iwant-style';
const Dark = styled.form`
${inputDark};
`;
...
<Dark action='' method=''>
<input type='search' placeholder='What are you looking for?' />
<button>Search</button>
</Dark>
...
Components
Comment

Usage
import styled from 'styled-components';
import { comment } from 'iwant-style';
const Comments = styled.div`
${comment}
`;
...
<Comments>
<section>
<h1>Petter</h1>
<h2>C0-Founder</h2>
<p>
Sit cupidatat commodo sit reprehenderit reprehenderit irure aliquip do
occaecat id.
</p>
</section>
<div>
<img
src='https://s3-us-west-2.amazonaws.com/s.cdpn.io/331810/profile-sample5.jpg'
alt='profile-sample5'
/>
</div>
</Comments>
...
Properties
Name |
Parameter |
Default |
${comment} |
|
default |
PreLoader

Usage
import styled from 'styled-components';
import { preloader } from 'iwant-style';
const Preloader = styled.div`
${preloader};
`;
...
<Preloader>
<div />
<div />
<div />
</Preloader>
...
Properties
Name |
Parameter |
Default |
${preloader} |
|
bubbles |
${preloaderCircle} |
|
circle |
Preloader Circle
import styled from 'styled-components';
import { preloaderCircle } from 'iwant-style';
const Preloader = styled.div`
${preloaderCircle};
`;
...
<Preloader>
<div />
</Preloader>
...
Avatar

Usage
import styled from 'styled-components';
import { avatar } from 'iwant-style';
const Avatar = styled.img`
${avatar};
`;
...
<Avatar src='https://s3-us-west-2.amazonaws.com/s.cdpn.io/331810/profile-sample9.jpg' />
...
Properties
Name |
Parameter |
Default |
${avatar} |
|
person |
Button

Usage
import styled from 'styled-components';
import { btn } from 'iwant-style';
const Button = styled.button`
${btn};
`;
...
<Button>Start</Button>
...
Properties
Name |
Parameter |
Default |
${btn} |
|
square |
${btn(param)} |
"disable" |
|
${btn(param)} |
"round" |
|
Input

Usage
import styled from 'styled-components';
import { input } from 'iwant-style';
const Input = styled.div`
${input};
`;
...
<Input>
<input type='text' required />
<span />
<label>Name</label>
</Input>
...
Dark
import styled from 'styled-components';
import { inputDark } from 'iwant-style';
const Dark = styled.form`
${inputDark};
`;
...
<Dark action='' method=''>
<input type='search' placeholder='What are you looking for?' />
<button>Search</button>
</Dark>
...
Properties
Name |
Parameter |
Default |
${input} |
|
animation |
Card

Usage
import styled from 'styled-components';
import { card } from 'iwant-style';
const Card = styled.div`
${card};
`;
...
<Card>
<h1>Card Title</h1>
<p>
Veniam elit commodo culpa sunt adincididunt nisi minim amet qui
sit pariatur occaecat. Veniam elitcommodo culpa sunt ad
incididunt nisi minim amet qui sitpariatur occaecat. sit pariatur
occaecat.
</p>
<hr />
<div>
<a href='#'>This is a link</a>
<a href='#'>This is a link</a>
</div>
</Card>
...
Properties
Name |
Parameter |
Default |
${card} |
|
info |
Pagination
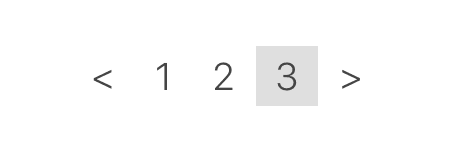
Usage
import styled from 'styled-components';
import { pagination } from 'iwant-style';
const Pagination = styled.ul`
${pagination};
`;
...
<Pagination>
<li>
<a href='#'><</a>
</li>
<li>
<a href='#'>1</a>
</li>
<li>
<a href='#'>2</a>
</li>
<li>
<a href='#'>3</a>
</li>
<li>
<a href='#'>></a>
</li>
</Pagination>
...
Properties
Name |
Parameter |
Default |
${pagination} |
|
arrow |
If you liked this project, contribute improvements or give a star ⭐️ on GitHub.
Thanks!
Top comments (0)