So, I've found a lot of crappy guides on the internet about how to integrate TailwindCSS with Angular 8, a lot of them involve some nasty hacks that I think are basically, shit. I've collated some information from various sources online to show you how I go about implementing TailwindCSS with Angular 8. If you're reading this and you don't know what TailwindCSS is, two things, one, where the hell have you been the past few years and two, go to tailwindcss.com and feast in the amazingness (is that even a word) that is Tailwind.
We'll be utilising the @angular-builders/custom-webpack package which will essentially allow us to tailor the webpack build to add tailwind into the build process.
First things first, install the following:
npm i tailwindcss postcss-scss postcss-import postcss-loader @angular-builders/custom-webpack -D
Next you need to open up your Angular project, I'm going to assume you're using sass, as well, why the fuck not?
Open your styles.scss
file and add the following at the top.
@import 'tailwindcss/base';
@import 'tailwindcss/components';
@import 'tailwindcss/utilities';
Next, you need to create the tailwind config file, to do so, open your terminal and smash in the following (inside the directory of your app of course).
npx tailwind init
Now we need to extend the webpack config, first, start by creating webpack.config.js
at the root of your project, this is what it should look like
module.exports = {
module: {
rules: [
{
test: /\.scss$/,
loader: 'postcss-loader',
options: {
ident: 'postcss',
syntax: 'postcss-scss',
plugins: () => [
require('postcss-import'),
require('tailwindcss'),
require('autoprefixer'),
]
}
}
]
}
};
Once you've added that, modify the angular.json
file to tell it to use the custom builder and config file.
{
"architect": {
"build": {
"builder": "@angular-builders/custom-webpack:browser",
"options": {
"customWebpackConfig": {
"path": "./webpack.config.js"
}
}
},
"serve": {
"builder": "@angular-builders/custom-webpack:dev-server",
"options": {
"customWebpackConfig": {
"path": "./webpack.config.js"
}
}
}
}
}
Now we're all up and ready to rumble, add some tailwind classes to an object and run
npm start
Extra
The whole point of Tailwind is to create custom utilities, you can do so by doing the following:
Modify your styles.scss
and add 2 new custom imports.
@import 'tailwindcss/base';
@import 'tailwindcss/components';
@import './custom-tailwind/custom-components.css'; // added
@import 'tailwindcss/utilities';
@import './custom-tailwind/custom-utilities.css'; // added
Make sure that they're added in the exact order above.
You can now create a folder inside the src directory called custom-tailwind
Inside this, you create the 2 files the same names as above, now you can create custom reusable utilities and components, for example:
custom-components.css
.btn {
@apply px-4;
@apply py-2;
@apply rounded;
@apply bg-indigo-500;
@apply text-white;
transition: 200ms;
}
.btn:hover {
@apply bg-indigo-600;
}
And now anywhere you want a button, just give it the class .btn
and it will apply all the tailwind classes.
For custom-utilities.css
, you can do the following:
.rotate-0 {
transform: rotate(0deg);
}
.rotate-90 {
transform: rotate(90deg);
}
.rotate-180 {
transform: rotate(180deg);
}
.rotate-270 {
transform: rotate(270deg);
}
And now anywhere, you can just call these classes. Neat huh!
Enjoy the power that is TailwindCSS + Angular 8!
Update:
I realise this is a repeated post, for some reason I had 3 dev.to accounts (god knows why) and this is the account I wanted to use, so I deleted this post along with the other account and re-created it here.
Update 2:
This also works the same way with Angular 9, I have this working using angular version "@angular/core": "~9.0.0",
Update 3: Angular 10.X
postcss-loader came with some breaking changes when it jumped from version 3 to 4.
I managed to get Angular 10 and Tailwind to play nicely by changing my webpack.config.js
to the following:
module.exports = {
module: {
rules: [
{
test: /\.scss$/,
loader: 'postcss-loader',
options: {
- ident: 'postcss',
- syntax: 'postcss-scss',
- plugins: () => [
- require('postcss-import'),
- require('tailwindcss'),
- require('autoprefixer'),
- ],
+ postcssOptions: {
+ ident: 'postcss',
+ syntax: 'postcss-scss',
+ plugins: [
+ require('postcss-import'),
+ require('tailwindcss'),
+ require('autoprefixer'),
],
},
},
},
],
},
};
Thanks to @phileagleson for spotting this!
You can also skip the entire above process and just the the Schematic that was created to do the work for you.
Note, you will still need to change the webpack.config.js
even if you use the Schematic when using this with postcss-loader
Version 4 and Angular 10
Update
Thanks to thieugiatri4492 for pointing out that if you used my manual method of installing Tailwind, and then moved over to use the schematic, you'll need to remove the webpack.config.js before using the schematic otherwise it will throw an error that it already exists!
Top comments (60)
Hi,
Is it possible to use tailwind @apply function inside of component specific style files? Instead of global one?
Currently my component html might become overloaded of classes and is harder to read.
Instead I could give component context meaningful classes to component HTML elements, and style them with @apply function inside of component stylesheet.
Do you know if it is possible?
Thanks, nice article 👍
As far as i know, I haven't found a way to use @apply inside a component specific style file. this is because webpack needs to know the file to compile into normal CSS.
There's nothing wrong with having loads of css classes inside HTML, some of mine are full of it, pointless making a class if im only going to use it once, or it's a specific instance i need styling. things like buttons and what not just go inside the custom-components.
If you do find a way of doing it, let me know!
Happy coding!
Did you find an answer to this? Without it Tailwind is unusable on any significant project.
I have used Tailwind on some Huge projects...
In fact, apart from some reusable items in my global styles.scss like
.btn
classes andinput
classes, all my "component" css files are empty... as i use the tailwind classes within the HTML. I have no need to write component specific styling.But you can indeed use @apply inside a component's style file, what am I missing? could you talk us through the issue you're facing please.
Unfortunately not, because tailwind is built through custom webpack process, adding component's styles to that is difficult and not supported.
I have actually just checked and this works fine for me, using scss, postcss-loader for webpack, and "tailwindcss": "^1.4.4".
dev-to-uploads.s3.amazonaws.com/i/...
sorry new here don't know why i cant attach photos.
Same for me. Works just fine when I've followed the implementation described in tis article.
Hi, I created a Schematic using this guide.
npmjs.com/package/@ikamva/ngx-tail...
I just tried this, and while it seemed to work just fine, it appears to have removed the configurations sections under build and serve in the angular.json file. I'm still learning, but is this expected or might I have done something wrong? I'm not sure if customWebpackConfig and configurations live and work in harmony, or if the former replaces the later.
I saw this too. Not sure if it's expected but you could probably add them back to the config yourself. What do you say @Sivuyile?
Thanks for writing the package, btw!
I have only just seen this. I've just tested it out and it works perfectly! Good work.
Mind if I fork it to add TailwindUI for those of us that use that too?
It's open-source, Feel free to do whatever you want.
Sorry late reply, I fixed that issue
Hi Sean, can I use both Angular Material and Tailwind CSS at the same time? I'm worried that it will bloat my project and some CSS utility classes might conflict? Have you tried it before?
I'd like to use Angular Material components and style them with Tailwind CSS. Thanks!
I do just that! I use material for things like Modals/Dialogs and Snackbars and Tailwind to style everything.
I don't actually include the Material CSS into my application!
This is encouraging but I haven't been able to get Angular/Material and Tailwind to work together with this config. It's possible I'm misunderstanding the build process in Angular 9.
If Tailwind is installed this way before running
add
for Material it warns you that you are not using the default builder and can't add Material.If you add Material before tailwind, it borks on
@import '~@angular/material/theming';
(which theadd
command adds for you) instyles.scss
withFailed to find '~@angular/material/theming'
.Can you share how you have your projects set up to get them to work together?
Oh I forgot to say. You need to add material first, double check all is working and then add tailwind.
Doing it the other way causes issues with webpack
I found the solution to this angular theme and tailwind conflict.
change '~@angular/material/theming' to either '~@angular/material/_theming.scss' or
'relative_path_to_node_module (../../node_modules/@angular/material/_theme.scss'
html.developreference.com/article/...
🖐
first, thanks a lot for your article!
i'm facing a problem after setting up custom-webpack
i just tried to implement this to an existing project but it seems that it creates error with previous dependencies (like @angular/material or flickity in my case).
Do I have to do something to import/include third party packages?
Example of an error
ERROR in ./src/app/orpheo/orpheo-presentation/orpheo-presentation.component.scss
Module build failed (from ./node_modules/postcss-loader/src/index.js):
ReferenceError: window is not defined
at Object. (C:\Users\Bams\Documents\plvbroker\plvbroker\node_modules\flickity\js\index.js:39:5)
at Module._compile (internal/modules/cjs/loader.js:776:30)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:787:10)
at Module.load (internal/modules/cjs/loader.js:653:32)
at tryModuleLoad (internal/modules/cjs/loader.js:593:12)
at Function.Module._load (internal/modules/cjs/loader.js:585:3)
at Module.require (internal/modules/cjs/loader.js:690:17)
at require (internal/modules/cjs/helpers.js:25:18)
at Object.plugins (C:\Users\Bams\Documents\plvbroker\plvbroker\webpack.config.js:14:23)
at Object.parseOptions (C:\Users\Bams\Documents\plvbroker\plvbroker\node_modules\postcss-loader\src\options.js:18:23)
at Promise.resolve.then (C:\Users\Bams\Documents\plvbroker\plvbroker\node_modules\postcss-loader\src\index.js:51:27)
at process._tickCallback (internal/process/next_tick.js:68:7)
Have you got the solution I having this problem
Error after ng serve
Web Config file
module.exports = {
module: {
rules: [
{
test: /.scss$/,
loader: 'postcss-loader',
options: {
ident: 'postcss',
syntax: 'postcss-scss',
plugins: () => [
require('postcss-import'),
require('tailwindcss'),
require('autoprefixer'),
]
}
}
]
}
};
// Don't import like this
@import '~@angular/material/prebuilt-themes/deeppurple-amber.css';
// Remove this ~
@import '@angular/material/prebuilt-themes/deeppurple-amber.css';
Data path "" should NOT have additional properties(customWebpackConfig).
Go to angular.json and modify builder in build and serve sections:
"projects": {
...
"example-app": {
...
"architect": {
...
"build": {
"builder": "@angular-builders/custom-webpack:browser"
"options": {
...
}
Then get error:
Schema validation failed with the following errors:
Data path "" should NOT have additional properties(browserTarget).
Found this when searching for this error. Just for reference for people who run into the same error in the future: I forgot to replace the default builder (@angular-devkit/build-angular) with @angular-builders/custom-webpack
Thanks, this helps me to install tailwind to an old Angular 8 app. Though I encounter several issues because the install script provided will install latest versions of its packages, thus breaks due to incompatibility with each other.
I added specific versions to correct them.
npm i tailwindcss@1.9.6 postcss-scss@3.0.4 postcss-import@12.0.1 postcss-loader@3.0.0 @angular-builders/custom-webpack@8.4.1 postcss@7.0.35 -D
This work with me in angular 8.
I have this problem when i try to start the app with ng serve. Can you please help me? :
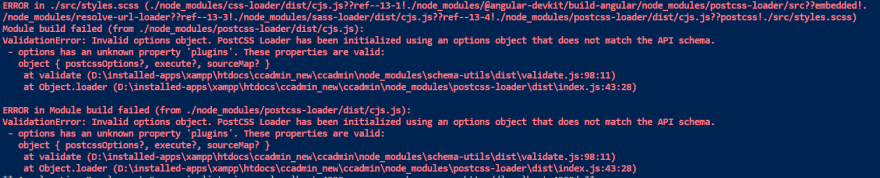
By any chance are you using Angular 10.x?
I think i have encountered the same issue when making a new application using angular 10, I didn't spend much time looking into fixing it. I was just playing with something...
I will update here once I have a working example and how i did it for Angular 10.
Yes, im using angular 10. I occured this problem , i think i have to revert to angular 9
Read the update to my guide. I changed it to work with Angular10
Now i face the problem with custom webpack builder, did you have occured this problem with angular 10?
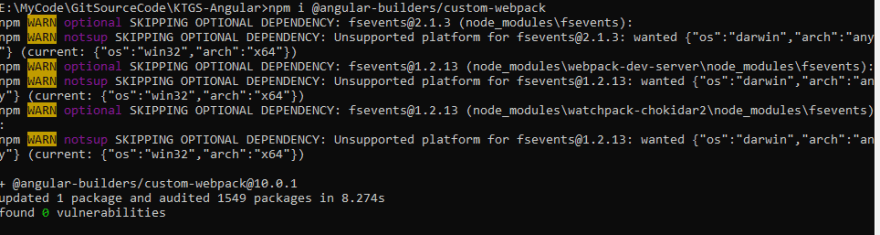

I have moved to use the schematic garygrossgarten/ngx-tailwind which i dont use a custom-webpack anymore, my angular.json looks like this
I suggest you try installing the schematic and go from there!
So, do i have to remove the custom webpack ? Or just install the schematic garygrossgarten/ngx-tailwind directly on my project?
You can try just installing the schematic. I’ve never tried undoing my own work in favour of the schematic.
If you do install it. All you should need to do is update the angular.Json to match mine
Thank you, i have successfully run with tailwind thank to your guide. But in your post, you have to warn people who already create webpack.config.js, remove it before install schematic because it will throw error that webpack.config.js already exist
Glad you got it to work!
I'm trying to follow this guide in a project using angular custom elements. Can't get it to work, the /postcss-loader is looking for my
styles.scss
in~projects/eddy-library/src/app/styles.scss
. But the correct director is without app, i.e.~projects/eddy-library/src/styles.scss
. How do I fix this?I think I'm missing something. I have the following code in my angular app.
In my angular app the grid doesn't work when I look at it in my dev inspector the grid classes don't seem to be there. The same code in a codepen and the class is there. So I'm assuming somehow the grid classes didn't get installed in my angular app. Other tailwind classes (ie bg-gray-400) are working. Anyone have any suggestions on how to fix this?
Code looks fine. What version of tailwind are you using? I’m using 1.2 and grids work fine like that.
From my package.json
New error in style.scss. This wasn't there before but I recopied and pasted from your post and now I'm getting this.
must have been something there. recompiled with an empty
custom-utilities.css
filewhile I'm getting the following waring.
It's compiling now and the grid is now working.
Hi,
After setting it with the custom webpack builder before using the schema is easy.
We implemented the apply via custom repository packages.
To make this work we modified the webpack config to also use the sass loader and url-resolver:
module.exports = {
module: {
rules: [
{
test: /\.scss$/,
use: [
{
loader: 'postcss-loader',
options: {
postcssOptions: {
ident: 'postcss',
syntax: 'postcss-scss',
plugins: [
require('postcss-import'),
require('tailwindcss'),
require('autoprefixer'),
],
},
},
},
"resolve-url-loader",
"sass-loader",
]
},
],
},
};
So in the style.scss we can add
@import 'tailwindcss/base';
@import 'tailwindcss/components';
@import 'tailwindcss/utilities';
/* You can add global styles to this file, and also import other style files */
@import "~@"; @import "~@....";