Originally published on bendyworks.com.
To finish off week two, I'm going to finally render some prim Unsplash birbs.
In PostItem
I'll use a Column
widget instead of the previous Card
widget. Card
creates a nice boarder shape for your material but now I don't want any shadows to draw attention away from the images.
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
ClipRRect(
child: Image.network(post.imageUrl),
borderRadius: BorderRadius.circular(10.0),
),
const SizedBox(height: 8.0),
Text(
post.username,
style: Theme.of(context).textTheme.headline,
),
const SizedBox(height: 8.0),
Text(
post.text,
style: Theme.of(context).textTheme.body1,
),
],
);
~~~{% endraw %}
{% raw %}`Column`{% endraw %}s are used to vertically arrange a number of widgets. In this case the image, the username, and the text. Along with those visible widgets there are also two {% raw %}`SizedBox`{% endraw %}es. These simply add a little space between the widgets. [{% raw %}`CrossAxisAlignment.start`{% endraw %}](https://docs.flutter.io/flutter/rendering/CrossAxisAlignment-class.html) is used to left align the text.
The image is wrapped in a [{% raw %}`ClipRRect`{% endraw %} widget](https://docs.flutter.io/flutter/widgets/ClipRRect-class.html) with a {% raw %}`borderRadious` to give it nice round corners. The `Text` widgets are using the [parent theme styling](https://flutter.io/docs/cookbook/design/themes) so they will stay current with future theme changes.
This is only a basic implementation of rendering images but I've planned some future improvements like [fading in](https://github.com/abraham/birb/issues/34) and [on device caching](https://github.com/abraham/birb/issues/35).
To add some space around all the `PostItems`, I'm updating {% raw %}`PostsList`{% endraw %} to use a [{% raw %}`Container`{% endraw %} widget](https://docs.flutter.io/flutter/widgets/Container-class.html) with some padding. I'm not putting the padding inside `PostItem` because it's the parents responsibility to position `PostItem`s in relation to each other. [`EdgeInsets.fromLTRB`](https://docs.flutter.io/flutter/painting/EdgeInsets/EdgeInsets.fromLTRB.html) will pad the left, top, right, and bottom edges respectively.
~~~dart
ListView(
children: items.map((Post post) {
return Container(
padding: const EdgeInsets.fromLTRB(16.0, 16.0, 16.0, 8.0),
child: PostItem(post),
);
}).toList(),
);
~~~{% endraw %}
In the image URLs, I've told Unsplash to return images with a width of 400px. This is simply to avoid downloading multi-megabyte images in development. Once users are uploading images in Birb, there will be more control over image sizes.
Now these are some prim birbs.
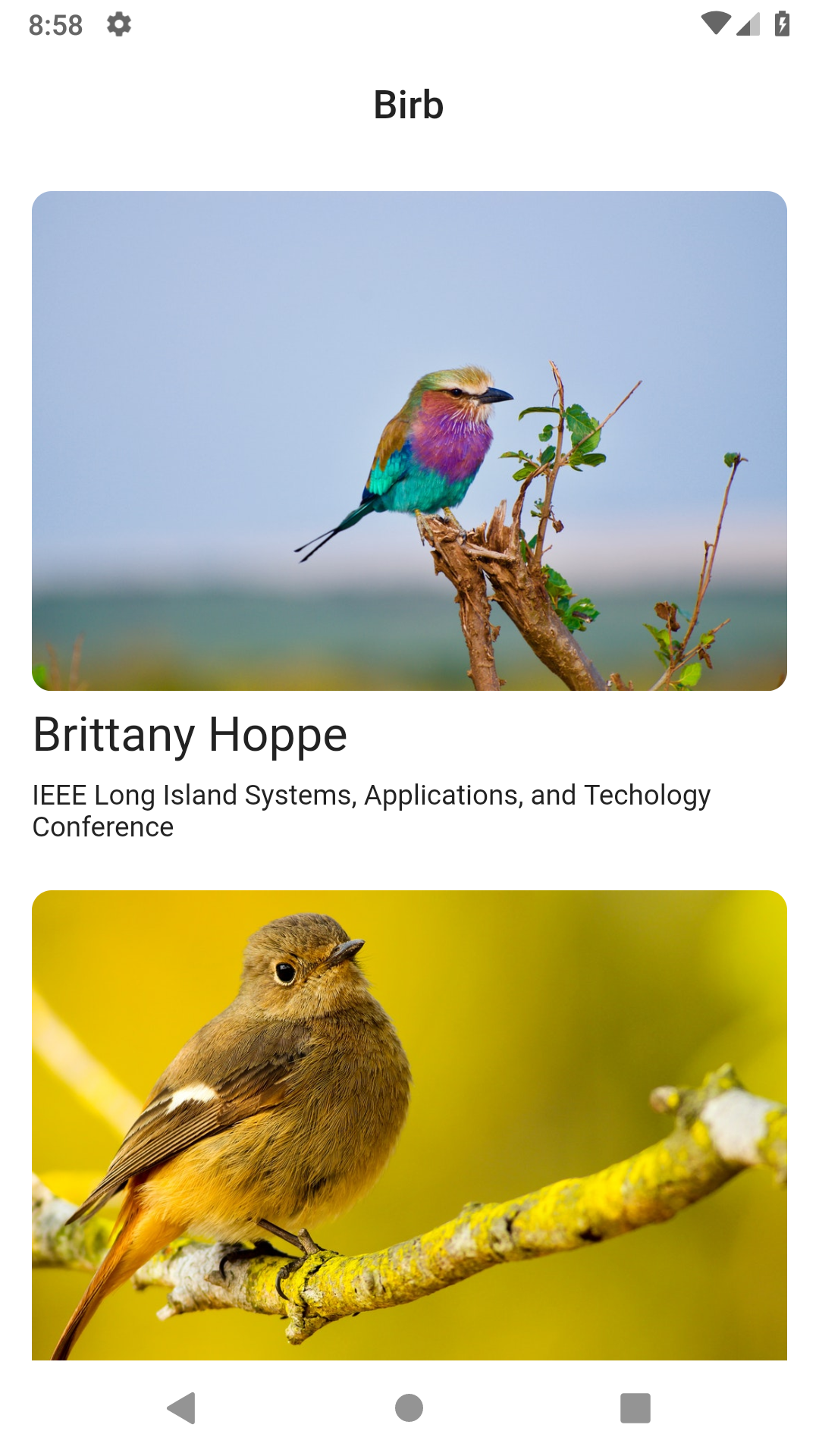
Flutter doesn't load images in tests, so those will have to be stubbed. There is an [easy to follow guide](https://iirokrankka.com/2018/09/16/image-network-widget-tests/) with instructions on using the [{% raw %}`image_test_utils` package](https://pub.dartlang.org/packages/image_test_utils) though.
## Code changes
- [#31 Render images and text](https://github.com/abraham/birb/pull/31)
Top comments (0)